-
- استكشف
-
How to Do Email Verification in PHP: A Comprehensive Guide
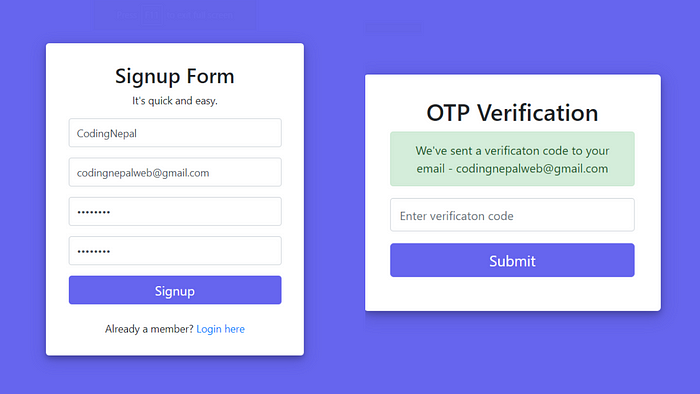
Email verification is one of the most essential aspects of web development, especially when handling user registrations or sensitive processes such as password resets. Ensuring that the email addresses provided by users are valid and functional can help reduce spam, ensure proper communication, and secure your website from fake or fraudulent accounts.
In this article, we’ll guide you through the process of email verification PHP, step-by-step. We’ll explore the importance of email verification, different methods, and provide a practical example of implementing it in PHP.
Why Email Verification Matters
Before diving into the technical aspects, let’s understand why email verification is so crucial:
-
Spam Prevention: Verifying emails ensures that the email addresses users enter are legitimate. This helps prevent spam, fake accounts, and bot registrations.
-
Secure Communication: By confirming that the email address provided is valid, you can communicate more effectively with users. For example, sending them a confirmation link or a password reset email.
-
Data Integrity: Email verification ensures that the data in your database is correct and authentic. Invalid emails or user errors are minimized, leading to a cleaner, more reliable system.
-
Boosts User Engagement: A verified email address often encourages users to check their inbox for important updates and follow through on account activities.
Methods of Email Verification in PHP
There are a few approaches to verifying an email address in PHP. Some of the most commonly used methods include:
-
Client-Side Validation (JavaScript): This method is used to provide instant feedback on the email format (like checking if the email contains "@" or "."). While it's great for user experience, it does not guarantee that the email address is real.
-
Server-Side Validation (PHP): This is where the real email verification happens. It checks whether the email address is syntactically correct and, in some cases, if the domain exists and accepts emails.
-
Email Verification with SMTP: This involves checking whether the domain is set up to accept emails (using an SMTP server). This is more robust than basic validation and requires more code.
-
Third-Party Email Verification Services: Some services, like NeverBounce or Hunter.io, offer APIs to validate email addresses by checking if the address exists and is active.
Implementing Email Verification in PHP
Let’s dive into how to validate email addresses in PHP. We will first show you a basic method to validate the email syntax and then an advanced way to check the domain using SMTP.
Step 1: Simple Email Validation
The simplest way to check if an email is valid is using PHP’s built-in filter_var()
function. This function checks the syntax of an email address to ensure it follows a proper format.
<?php
$email = "test@example.com";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Valid email address.";
} else {
echo "Invalid email address.";
}
?>
This code checks if the provided email address follows the basic rules of an email format (i.e., contains "@" and a domain). However, this method only checks the format and does not confirm that the email exists.
Step 2: Domain Verification Using PHP
To go beyond basic syntax checking and verify if the domain of the email address exists, you can use PHP's checkdnsrr()
function. This function checks if the domain has any valid DNS records (like MX records) that indicate the ability to receive emails.
<?php
$email = "test@example.com";
$domain = substr(strrchr($email, "@"), 1);
if (checkdnsrr($domain, "MX")) {
echo "Domain exists and can receive emails.";
} else {
echo "Domain does not exist or cannot receive emails.";
}
?>
In this example, we extract the domain from the email address and use checkdnsrr()
to confirm whether the domain has MX (Mail Exchange) records set up, which indicates that the domain is capable of receiving emails.
Step 3: Sending a Verification Email
Once you’ve validated the email address, the next step is to send a verification email to the user. This email will contain a unique verification link that the user must click to confirm their email address.
Here’s how you can send an email in PHP using the mail()
function:
<?php
$to = "user@example.com";
$subject = "Email Verification";
$message = "Please click on the link below to verify your email address:\n";
$message .= "http://yourdomain.com/verify?email=user@example.com";
$headers = "From: no-reply@yourdomain.com";
if (mail($to, $subject, $message, $headers)) {
echo "Verification email sent successfully!";
} else {
echo "Failed to send verification email.";
}
?>
In this example, the user receives a link that they must click on to verify their email address. This is usually handled by a special page on your website that checks the provided email and marks it as verified in your database.
Step 4: Using SMTP to Verify Email Addresses
For a more advanced approach, you can connect to the email server using SMTP to verify the email address. This step checks whether the email can actually be delivered to the recipient's mailbox.
<?php
function checkEmailExists($email) {
$domain = substr(strrchr($email, "@"), 1);
$mxhosts = [];
if (getmxrr($domain, $mxhosts)) {
$connection = fsockopen($mxhosts[0], 25, $errno, $errstr, 5);
if ($connection) {
fwrite($connection, "HELO $domain\r\n");
fwrite($connection, "MAIL FROM:<test@yourdomain.com>\r\n");
fwrite($connection, "RCPT TO:<$email>\r\n");
fwrite($connection, "QUIT\r\n");
$response = fgets($connection, 1024);
if (strpos($response, "250") === 0) {
echo "Email address is valid!";
} else {
echo "Email address is invalid!";
}
fclose($connection);
}
} else {
echo "Domain does not have MX records!";
}
}
checkEmailExists("test@example.com");
?>
This example connects to the email server and sends an SMTP command to check if the email can be received. If the server responds positively, the email address is considered valid.
Conclusion
Implementing email verification in PHP is an essential practice to ensure that users provide valid and legitimate email addresses during registration or other processes. By validating the syntax, checking the domain, and sending a verification email, you can enhance the security and reliability of your system.
While simple validation is a good start, advanced methods like SMTP checks offer a more thorough verification process. Regardless of the method you choose, ensuring proper email validation can help prevent spam, enhance user experience, and maintain data integrity on your website.